Writing To The E-ink Display With Micropython
I’d gotten as far as running a few lines of code on the Pico, so the next thing was to work out if I could display anything at all on the e-ink display. Since I’d already messed up by buying the wrong HAT type, I still had a small doubt about whether I would get the two to work together at all.
Waveshare has its own Github respository so I headed there to track down the correct Python file. I knew I needed one of the files that started with “Pico_ePaper-2.13-“, but which one?
There was a little stick on the display telling me I had a “v3”, but working out whether I had an A, B, C or D model was a mixture of guesswork and deduction. The file for both B and C appeared to support multiple colours, which is not what I had, so it wasn’t those. I had nothing else to go on, so I tried both A and D. Neither seemed to work properly, although I did get the screen to flicker a bit so something was happening.
I can’t even remember how I finally got the thing working, but it appears to be through a hybrid of files that started as Pico_ePaper-2.13-B_V4.py with all the code that referred to the red image data removed. The whole process was made more difficult by the fact that I seemed to have messed up connecting two of the pins, having not spotted that there was a pin labelled “GND” right between “GP21” and “GP22” on the Pico board.
This was my “driver” module:
from machine import Pin, SPI
import framebuf
import utime
EPD_WIDTH = 122
EPD_HEIGHT = 250
RST_PIN = 12
DC_PIN = 8
CS_PIN = 9
BUSY_PIN = 13
class EPD:
def __init__(self):
self.reset_pin = Pin(RST_PIN, Pin.OUT)
self.busy_pin = Pin(BUSY_PIN, Pin.IN, Pin.PULL_UP)
self.cs_pin = Pin(CS_PIN, Pin.OUT)
if EPD_WIDTH % 8 == 0:
self.width = EPD_WIDTH
else :
self.width = (EPD_WIDTH // 8) * 8 + 8
self.height = EPD_HEIGHT
self.spi = SPI(1)
self.spi.init(baudrate=4000_000)
self.dc_pin = Pin(DC_PIN, Pin.OUT)
self.buffer_black = bytearray(self.height * self.width // 8)
self.init()
def digital_write(self, pin, value):
pin.value(value)
def digital_read(self, pin):
return pin.value()
def delay_ms(self, delaytime):
utime.sleep(delaytime / 1000.0)
def spi_writebyte(self, data):
self.spi.write(bytearray(data))
def module_exit(self):
self.digital_write(self.reset_pin, 0)
# Hardware reset
def reset(self):
self.digital_write(self.reset_pin, 1)
self.delay_ms(50)
self.digital_write(self.reset_pin, 0)
self.delay_ms(2)
self.digital_write(self.reset_pin, 1)
self.delay_ms(50)
def send_command(self, command):
self.digital_write(self.dc_pin, 0)
self.digital_write(self.cs_pin, 0)
self.spi_writebyte([command])
self.digital_write(self.cs_pin, 1)
def send_data(self, data):
self.digital_write(self.dc_pin, 1)
self.digital_write(self.cs_pin, 0)
self.spi_writebyte([data])
self.digital_write(self.cs_pin, 1)
def ReadBusy(self):
print('busy')
while(self.digital_read(self.busy_pin) == 1):
self.delay_ms(10)
print('busy release')
self.delay_ms(20)
def TurnOnDisplay(self):
self.send_command(0x20) # Activate Display Update Sequence
self.ReadBusy()
def TurnOnDisplayPart(self):
self.send_command(0x20)
self.ReadBusy()
def SetWindows(self, Xstart, Ystart, Xend, Yend):
self.send_command(0x44) # SET_RAM_X_ADDRESS_START_END_POSITION
self.send_data((Xstart>>3) & 0xFF)
self.send_data((Xend>>3) & 0xFF)
self.send_command(0x45) # SET_RAM_Y_ADDRESS_START_END_POSITION
self.send_data(Ystart & 0xFF)
self.send_data((Ystart >> 8) & 0xFF)
self.send_data(Yend & 0xFF)
self.send_data((Yend >> 8) & 0xFF)
def SetCursor(self, Xstart, Ystart):
self.send_command(0x4E) # SET_RAM_X_ADDRESS_COUNTER
self.send_data(Xstart & 0xFF)
self.send_command(0x4F) # SET_RAM_Y_ADDRESS_COUNTER
self.send_data(Ystart & 0xFF)
self.send_data((Ystart >> 8) & 0xFF)
def init(self):
print('init')
self.reset()
self.ReadBusy()
self.send_command(0x12) #SWRESET
self.ReadBusy()
self.send_command(0x01) #Driver output control
self.send_data(0xf9)
self.send_data(0x00)
self.send_data(0x00)
self.send_command(0x11) #data entry mode
self.send_data(0x03)
self.SetWindows(0, 0, self.width-1, self.height-1)
self.SetCursor(0, 0)
self.send_command(0x3C) #BorderWavefrom
self.send_data(0x05)
self.send_command(0x18) #Read built-in temperature sensor
self.send_data(0x80)
self.send_command(0x21) # Display update control
self.send_data(0x80)
self.send_data(0x80)
self.ReadBusy()
return 0
def display(self):
self.send_command(0x24)
for j in range(0, self.height):
for i in range(0, int(self.width / 8)):
self.send_data(self.buffer_black[i + j * int(self.width / 8)])
self.TurnOnDisplay()
def Clear(self, colorblack):
self.send_command(0x24)
for j in range(0, self.height):
for i in range(0, int(self.width / 8)):
self.send_data(colorblack)
self.TurnOnDisplay()
def sleep(self):
self.send_command(0x10)
self.send_data(0x01)
self.delay_ms(2000)
self.module_exit()
I haven’t yet managed to track down what all the send_command statements actually do, but I feel sure there must be some documentation for them somewhere.
Anyway, finally, I had the equivalent of “hello world” on the display, without really knowing how. Also, I had discovered that the epaper display naturally preferred to display in portrait mode, which wouldn’t work for the layout I had in mind for my weather display.
epd = EPD()
epd.Clear(0xff)
epd.imageblack = framebuf.FrameBuffer(epd.buffer_black, epd.width, epd.height, framebuf.MONO_HLSB)
epd.imageblack.fill(0xff)
#epd.imagered.fill(0xff)
epd.imageblack.text("Waveshare", 0, 10, 0x00)
epd.imageblack.text("ePaper-2.13-B", 0, 25, 0x00)
epd.imageblack.text("RPi Pico", 0, 40, 0x00)
#epd.imagered.text("Hello World", 0, 55, 0x00)
epd.display()
epd.delay_ms(2000)
epd.imageblack.vline(10, 90, 40, 0x00)
epd.imageblack.vline(90, 90, 40, 0x00)
epd.imageblack.hline(10, 90, 80, 0x00)
epd.imageblack.hline(10, 130, 80, 0x00)
epd.imageblack.line(10, 90, 90, 130, 0x00)
epd.imageblack.line(90, 90, 10, 130, 0x00)
epd.display()
epd.delay_ms(2000)
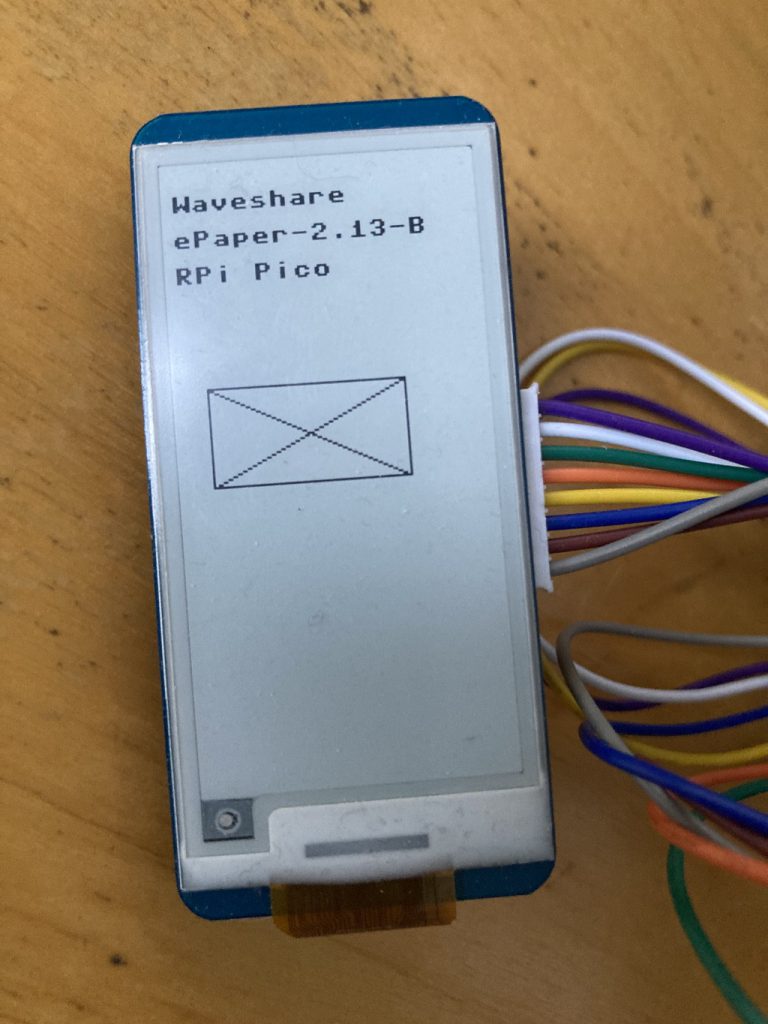
It felt like I’d conquered most of the unknowns anyway, so now onto showing some actual weather.